Computer Science Guide For Self Taught Developers
Posted on February 21, 2025
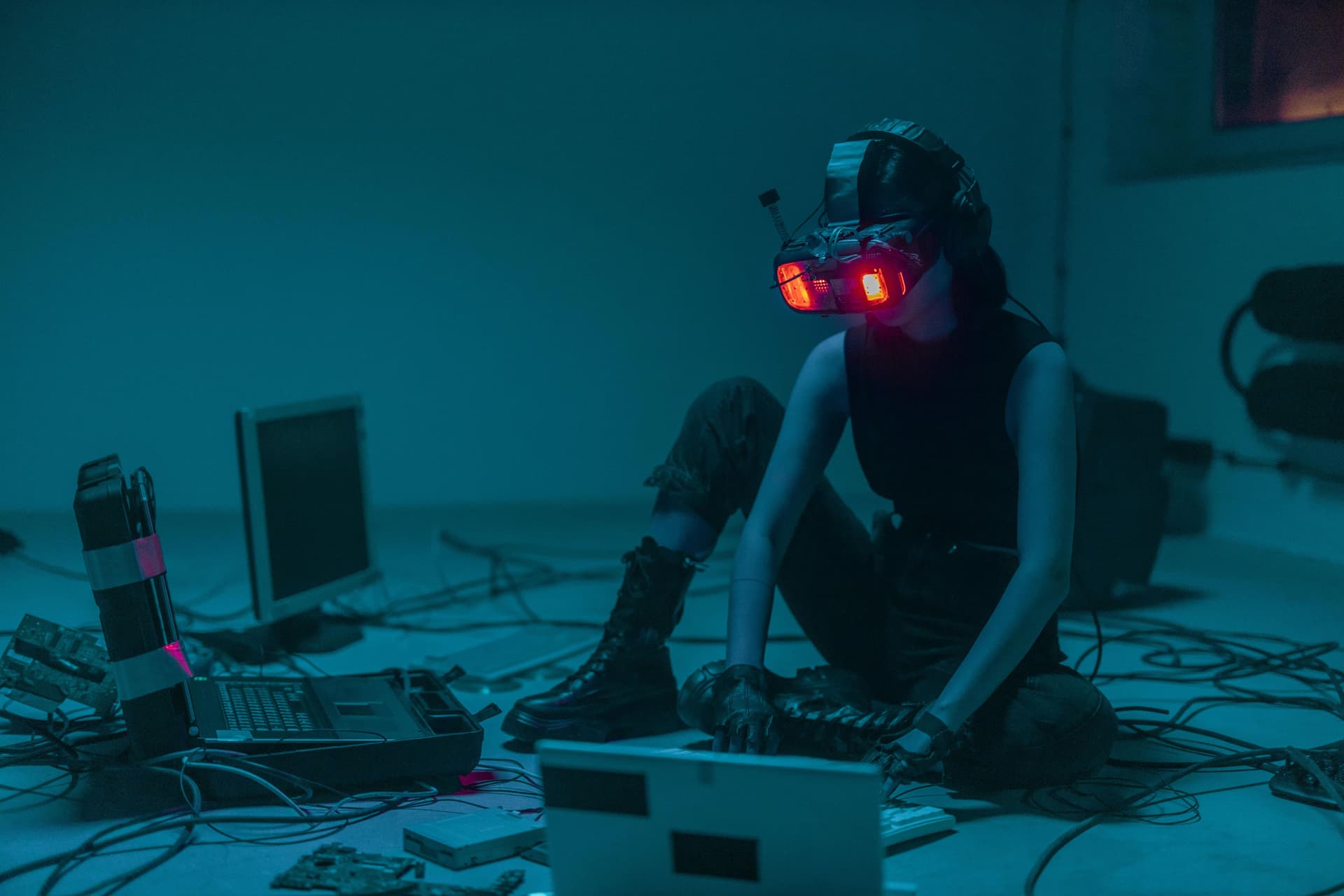
Software Engineering Self-Study Curriculum (Beginner to Advanced)
Introduction
This roadmap is designed for aspiring software engineers without a formal CS degree. It covers all the essential topics from the ground up, using free resources (online courses, books, tutorials) and a progressive learning path.
The journey starts with core programming skills, then builds up the required mathematics, followed by data structures and algorithms, fundamental computer science concepts (like operating systems, networking, databases, system design), and finally supplementary topics (security, DevOps, AI/ML, software architecture, cloud).
Each stage builds on the previous ones, ensuring you solidify basics before moving to advanced topics. You can follow this sequence step-by-step while practicing and building projects at each stage to reinforce your learning.
Below is the detailed curriculum roadmap with explanations of why each subject is necessary, what it teaches you, and how it applies in real-world software engineering.
1. Programming Fundamentals (Beginner to Advanced)
Course Recommendations
Start with an introductory programming course in a beginner-friendly language (like Python or JavaScript) to grasp basic coding:
- Harvard CS50x – Introduction to Computer Science (edX): A famous beginner course that teaches how to think algorithmically and solve problems efficiently, covering basics in C, Python, web, etc.
- MIT OpenCourseWare – Introduction to Computer Science and Programming in Python: Introduces computational thinking using Python.
- Automate the Boring Stuff with Python by Al Sweigart: A free online book that teaches Python programming through practical projects (great for absolute beginners to get hands-on practice).
- FreeCodeCamp or Codecademy (Free tracks): Interactive coding exercises in languages like JavaScript or Python for additional practice.
Why It’s Necessary
Programming is the core skill of software engineering – it’s how you instruct the computer to perform tasks. No matter what area of software development you go into, you must be able to write and understand code. A strong foundation in programming allows you to:
- Build software
- Troubleshoot problems
- Implement concepts like data structures and algorithms
What It Teaches
- Syntax and Basics: Writing correct code (variables, data types, loops, conditionals, functions)
- Problem-Solving: Thinking like a programmer – breaking down problems into step-by-step solutions (algorithms) and translating them into code
- Fundamental Concepts: Abstraction, algorithms, data structures, encapsulation, and basics of memory management
- Multiple Paradigms: Structured programming, object-oriented programming, and basics of functional programming
2. Essential Mathematics for Software Engineering
Course Recommendations
Use free courses and books targeted at specific math topics:
- Discrete Mathematics / Math for CS: Mathematics for Computer Science (MIT OpenCourseWare)
- Linear Algebra: MIT 18.06 Linear Algebra (Gilbert Strang)
- Probability & Statistics: Khan Academy’s Probability and Statistics and Think Stats by Allen Downey
- Calculus: Khan Academy – Calculus
Why It’s Necessary
Computer Science is “a runaway branch of applied math.” These math fundamentals are necessary for:
- Understanding algorithms
- Graphics, cryptography, machine learning
- Performance optimization in software
3. Data Structures & Algorithms
Course Recommendations
- Princeton Algorithms (Coursera)
- MIT Introduction to Algorithms (OCW)
- The Algorithm Design Manual by Steven Skiena
- LeetCode, HackerRank, CodeSignal for interactive coding practice
Why It’s Necessary
- Data structures and algorithms determine the efficiency of software
- Understanding them is required for technical interviews
- Helps in writing scalable and high-performance applications
4. Computer Science Foundations (Systems & Core Concepts)
Topics & Course Recommendations
- Operating Systems: Operating Systems: Three Easy Pieces (OSTEP)
- Networking: Stanford CS144: Introduction to Computer Networking
- Databases: SQLBolt, Introduction to Databases by Jennifer Widom (Stanford)
- System Design: System Design Primer (GitHub)
Why It’s Necessary
- Understanding operating systems helps in debugging, memory management, and process handling
- Networking is crucial for web development, APIs, and distributed systems
- Knowing database design improves performance and data integrity
- System design enables building scalable software architectures
5. Supplementary Topics (Advanced & Specialized Skills)
Topics & Course Recommendations
- Cybersecurity: CS50’s Introduction to Cybersecurity
- DevOps: Codecademy’s Introduction to DevOps
- AI/Machine Learning: Andrew Ng’s Machine Learning (Coursera)
- Software Architecture: Software Design and Architecture (Coursera)
- Cloud Computing: AWS Cloud Practitioner Essentials
Why It’s Necessary
- Cybersecurity: Protecting software from vulnerabilities
- DevOps: Automating CI/CD pipelines, infrastructure as code
- AI/ML: Understanding machine learning basics enables automation and smart applications
- Software Architecture: Designing scalable, maintainable applications
- Cloud Computing: Deploying software at scale with AWS, GCP, or Azure
Conclusion
By following this curriculum, you’ll transition from a beginner to an advanced software engineer with a broad and deep skill set.
- Apply knowledge by building projects
- Join coding communities & contribute to open source
- Practice coding challenges
- Be patient, persistent, and curious
With dedication, you will become a professional software engineer capable of designing, building, and scaling real-world software applications.
🚀 Good luck on your learning journey!